Notes from: Introduction to Programming
This guide uses the Java language to cover programming topics.
1. Introduction to programming
Programming is important.
2. Class vs Objects
Class - is a blueprint or outline. A class manages state and behavior.
Object - is an instance of a class. An object is what lives in memory. It is the house made from the blue print.
//define a class by using "class"
class SomeClass {
//stuff
}
//create an object by using "new"
SomeClass sc = new SomeClass();
Class constructors - "a special type of subroutine called to create an object. It prepares the new object for use, often accepting arguments that the constructor uses to set required member variables." - wikipedia
Class contructor - "a special method that is used to initialize objects. The contructor is called when an object of a class is created. It can be used to set initial values for object attributes." - w3schools
3. Constructors
Invoking the keyword new starts a process called instantiation.
Instantiation is the process of Java creating an instance of an object in memory according to the class type
Instantiation is like spawing an object. You can spawn many different houses from the same blueprint!
A constructor is a block of code that is executed when the new keyword is invoked.
The default no-arg constructor is created by the compiler if you don't write any constructors.
class A{
//contructor
public A(){...}
}
If you write your own constructor, then the compiler will NOT create a default no-arg constructor for you.
class SomeClass {
int somevariable;
public static void main(String[] args){
...
}
}
its possible to overload a constructor if you specify different parameters for each constructor.
4. Encapsulation
What is Encapsulation?
Encapsulation - is the act of enclosing an item inside of a capsule or container.
In software development, encapsulation refers to the act of protecting data members from outside influence.
Data hiding is an oversimplification of the concept of encapsulation; it is partially correct.
Data hiding refers to the act of declaring member variables private, so that outside classes and other entities cannot directly access these variables.
This is a partial implementation of encapsulation, but doesnt completely describe the term in that with encapsulation you don't have to use a private modifier only.
There are others such as protected or default access levels that languages use to restrict access to data.
5. Access Modifiers
We are going to explore more about the public keyword on the main method. It is part of a group of keywords known as access modifiers. There are three of them and each controls how other classes access or call a method variable or class.
What is an access modifier?
An access modifier - changes the permission levels other classes have to this method, class or variable.
An access modifier - is a keyword that you can apply to classes, methods, and instance variabels to control whether other classes are permitted to manipulate it.
There are 3 access modifiers, but there are 4 levels of access listed from least restrictive to most restrictive:
-
public - means that all classes in any package are available to call or execute this method.
least restrictive; all classes have access
-
default - not an access modifier;
only classes in the same package have access
-
protected - Only subclasses (in any package) have access;
classes in same package have access as well
-
private - most restrictive;
no classes (except inself) have access
Using an Access Modifiers
Typically, when you declare a variable or method, you'll specify an access modifier in front of it.
Classes only support the public or default level of access.
Methods and variables support all access modifiers.
Common patterns create private varaibles and public accessor methods.
Rule of thumb: Use the most restrictive access level unless you absolutely need the access.
6. Inheritance
Inheritance - Gives access to the variables and methods of one class to another.
Inheritance creats an "is-a(n)" relationship between the child and parent class. But the converse is not always true.
This relationship is one-way. All magazines are books, but not all books are magazines.
If one object is an example or a subtype of another object, the two can likely be arranged as an inheritance relationship.
For example:
- a tiny home (child class) is-a type of house (parent class).
- an apple (child class) is-a type of fruit (parent class).
- a warrior (child class) is-a type of hero (parent class).
- a square is-a type of rectangle.
7. Polymorphism
Polymorphism means many forms and reffers to the is-a relationship between two objects create by ineritance.
Is-A(n) Relationships
When one class extends another, an instance of that child class is also an instance of the parent class.If one class can be described as an exmaple or subtype of another class it can probably inherit from it.
Contractual Members
A child class is guaranteed to have the public variables and methods of a super class, even if the implementations differ
This lets you write code that expects instances of a super class, but can also handle instances of child classes.
References Review
Objects are stored in heap memory, and their addresses are stored in reference variables.
reference variables use a class as a type - they can hold the address of any object that is-an instance of that class.
Dog myDog = new Dog();
Creates a new Dog object, stores the address of the Dog object in a reference variable named "myDog" that is type Dog
Reference types.
Upcasting
Method Overloading
At times you may need to have functionality in a class that supports similar types of input and output.
Instead of creating methods with really similar or unquie names, what you really need to do is employ method overloading to make your class more readable.
What is method overloading?
Method Overloading - is the creation of methods with the same name as another in the same class but it differs in parameters.
Example:
class Claculator
{
public int add(int i, int j)
{
return i + j;
}
public double add(double i, double j)
{
return i + j;
}
public long add(long i, long j)
{
return i + j;
}
}
Overridding Methods
Inheriting Methods
A method signature is the basic outline of a method:
Ex: modifiers methodName(parameters) exceptions
Any child class that you write to extend a class will inherit its methods as long as they are not marked private.
A child class can specify a new implementation for a method that is inherited.
Significance of Overriding
Overriding allows child classes to provide new implementation for their parent functionality. Useful for polymorphic references.
To override a method, you must first have a method on a parent class. In the child class, you will need to specify a mehtod of the same signature.
8. Abstraction
Abstraction - occurs when you simplify a complex thought or complex system.
When designing something, it is possible to simplfy a complex system by drawing a "black box" around the complexity and focus on the inputs and outputs of the system.
Indeed, it is sometimes helpful to break complex systems down into a network of black boxes.
The black box is an abstraction of the system.
Interfaces
An interface is a special type of construct in Java.Used to guarantee the availability of methods in implementing classes.
All mehtods are: public abstract
All variables are public static final
Intrefaces are written similar to classes except they use the word "interface" instead of the word "class".
public interface Doable {}
public class Chore
extends Task
implements Doable{}
Interfaces make compiler-enforced contracts. A class that implements an interface must
A class that implements an interface must provide an implementation for every interface method, or be an abstract class
Interface methods do not have implementations and interface variables are static, so no states or behaviors are inherited
Said to have "type" inheritance. A class that implements an interface is polymorphically considered an instance of the interface.
Name interfaces for adjectives or verbs.
An interface describes what behaviors a class should have, it provides none of its own.
Ex. Runnable, Serializable, Functional
A class can implement multiple interfaces
Interfaces extend other interfaces, with no limits on the quantity allowed
Interfaces cannot extend classes, and do not implement other interfaces
Keyword:static
The static keyword determines if a method or variable belongs to the class as a whole or only an instance. What is Static?Static - is a keyword that marks an item as a member of the class.
Recall that a class acts as a blueprint and an object is an instance of the class.
Any methods or variables that you label as static are available without having to create objects of that class type.
That main method is always labeled as static.
Keyword:final
What is Final?Something marked final means that it cannot change its value.
If a variable is marked final, then code cannot change its value once set.
If a method is marked final, then code in subclasses cannot override it.
If a class is marked final, then another class cannot extend it.
9. Loop Statements
What is a while loop?
A while loop will repeat a block of code based on a condition; it essentially tells the program to start back up at the top from where it is defined.
while (condition)
{
...
}
What is a Do-while loop?
A do-while loop, will execute its statements at least once, and then continue repeating them based on a condition.
do
{
...
} while (condition);
10. Data Structures
Variables are not sufficient to organize data.
Data structures are used to organize data.
Arrays - a linear collection of variables of same size, and often type.
Arrays are core to most languages.
- Arrays reserve memeory so their contents are adjacent.
- Array sizes are fixed.
- Array contents are accessed by an index.
- Array indices start at 0 in almost all languages.
- Removed array elements leave an empty index.
- Arrays must always be of a fixed length and can only be specified once.
Vectors or Lists are:
- flexible in size.
- Memory is not reserved at initialization.
- Often just a wrapper around an array.
- Can access elements by an index.
- Internaly doubles in size when full.
- Adding or removing elementscauses later elements to shift.
- A linear collection without an index
- Made of Nodes
- A Node is a wrapper for the actual element, with a link to the element
- Accessing a particular element means searching the list
- Adding or removing an element means linking to neighbors
- A collection of Nodes
- Each node wraps the element, and tracks an assigned key for that element
- The map must be able to return a node when given the approapriate key
11. Exceptions
Exceptions
- Problems that occur during compilation or execution of a program
- Exceptions are thrown and can be caught and handled independently
- Checked:
Detected during comilation - Unchecked:
Occur during runtime
- A method declarations can have a throws clause, indicating that it may throw and will not handle certain exceptions.
- A method with a throws clause is a source for checked exceptions.
11. SQL DB
Data Organization
- Companies need a way to quickly search records.
- Users need to be able to access records concurrently.
- Users need to always have the most recent data.
- Related data should be linked.
- Link data in one table to related data in another table.
- Tables are like named spreadsheets that each track a single idea or type of record.
- Columns are the properties of each record.
- Each row is itself a record.
- A standard for database languages.
- Managed by the American national Standards Institute (ANSI).
- Databases often implement the SQL standard differently, or add to it.
- SQL is easy to read by design.
- Interaction occurs through SQL statements or "queries."
- DDL: Data Definition Language Statements that define database structure.
- DML: Data Manipulation Language Statements that manipulate table data.
- TCL: Transaction Control Language Statements that control the execution of queries.
CRUD: Create, Read, Update, Delete.
Transaction: A group of SQL statements executed together.
- VARCHAR: text data of variable length
- INTEGER: 32-bit, signed whole numbers
- SMALLINT: 16-bit, signed whole numbers
- NUMBER(n,d): Decimal numbers with limited significant figures
- FLOAT: floating-point numbers
- DATE: A day/month/year record
- TIMESTAMP: A combination of a date and time
Column attributes that restrict data
- PRIMARY KEY: A column whose values uniquely identify each row
- Composite Key: Two or more columns acting together as a primary key
- FOREIGN KEY: A column in one table that references a column in another table
- UNIQUE KEY: A column whose values must be unique in each row
- NOT NULL: The column must have a value in each row.
- CHECK: The column values must meet some criteria
A Join is an operation that combines the results of two tables.
Common types of joins:
- A left join or left outer join returns the results from the frist table and records from the second table that match on a specified condition.
- A right join will return the results of the second table and records from the first only if they match records in the second based on a condition.
- An inner join will return only records from both tables that match based on a condition.
- A full join or full outer join will retrun all records from both tables.
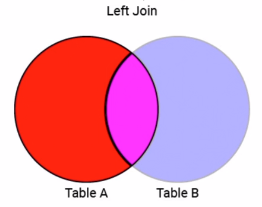
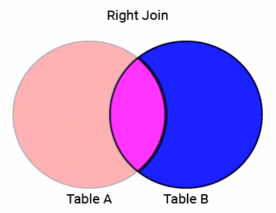
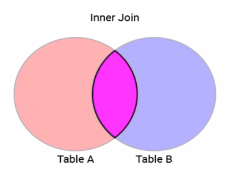
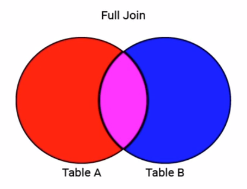
Basic Sytax
A WHERE clause is appended to the end of a SELECT statement.
SELECT STATEMENT WHERE condition;
The condition indicates a column to filter and any comparison operators and values.
Ex:
SELECT * FROM EMPLOYEES WHERE
employee_id > 5;
12. HTML/CSS
HTML
- Hypertext Markup Lanuage
- Used to build webpages and provides structure
- Hypertext: content linked to related content
- Tags surround and "mark up" text, forming an element
<!DOCTYPE html>
<html>
<head>
<title>My First Webpage</title>
</head>
<body>
<p>A paragraph</p>
</body>
</html>
Element - a set of tags and their enclosed content. Everything higlighted is part of the element: <tag>content</tag>
Highlighted is an example of a paragraph element:
<p>We went to the park.</p>
Tags:
Highlighted is an example of a paragraph tag:
<p>We went to the park.</p>
Some tags are composed of both an opening tag and closing tag:
<tag attribute="value">content</tag>
A tag can also be a self closing tag:
<selfclosingtag/>
Attributes examples:
- <img src="logo.png" />
- <p class="first" style="color:grey">We went to the park.</p>
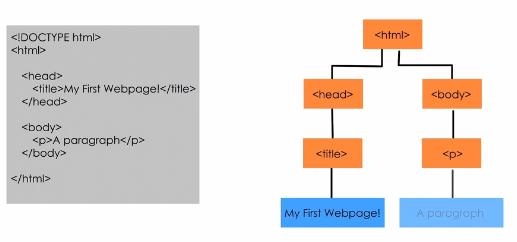
Tables
Webpage design naturally fits a grid system:
- <table>: the table element begins with the table tag
- <tr>: table row
- <td>: table data
- <th>: table header
- <thead>: table head
- <tbody>: table body
- <tfoot>: table footer
- <ol>: ordered list creates an itemized list.
- <ol type="a">: renders lower case letters
- <ol type="A">: renders upper case letters
- <ol type="i">: renders lower case letters Roman numerals
- <ol type="I">: renders upper case letters Roman numerals
- <ul>: unordered list creates a bulleted list.
- <li>: list item denotes an item in any kind of list.
- Deals with designing web pages to display differently according to the device.
- Users prefer to scroll vertically than horizontally due to phones and scroll wheels.
- Design for the smallest size first; increase afterwards.
- Lines of text should be 8 to 10 words long.
- Use CSS Media Queries to apply styling to different device sizes
- specify min-width property
- use relative dimensions
- Avoid @import statements
- <link rel="stylesheet" href="main.css"> :applies to all device sizes
- <link rel="stylesheet" media="(max-width:600px)" href="mobile-small.css">
- <link rel="stylesheet" media="(min-width:601px)" href="mobile-large.css">
- Do NOT use large fixed width elements
- Avoid the use of <table> for layout
- Use relative width values
- Use <div> for layout
CSS Syntax
Summary- Block-style - surround portions of code in curly braces.
- Selector - identifies what element to style.
- Property - identifies which component to change.
- Value - specifies the value of the attribute.
- Declaration - indicates a property/value pair.
{
property:value;
}
p
{
color:red;
}
CSS Selectors
Types of Selectors- element
- class .
- id #
- all *
- multiple, elements
- parent > child
- ancestor descendant
- sibling+sibling2
- predecessor~successor
Selects a specific tag
-
p
{
//apply CSS here
}
- Selects all elements with the specified class attribute
- Use a . before the class name
-
.purple
{
//apply CSS here
}
- Selects all elements with the specified id attribute
- Use the pound symbol (#) before the id name
-
#banner
{
//apply CSS here
}
- Selects al elements on the page
- use the asterisk symbol (*)
-
{
//apply CSS here
}
- Selects multiple elemetns using different selectors
- Use the comma (,) to delimit multiple selectors
-
html, body, p
{
//apply CSS here
}
- Selects all immediate matching children of an element
- Use the greater than symbol (>) between the parent and child
-
div > p
{
//apply CSS here
}
- Selects all matching descendants of an element
- Use a space ( ) between the ancestor and descendant
-
div p
{
//apply CSS here
}
- Selects all matching siblings of an element
- Use the plus symbol (+)
-
h3 + p
{
//apply CSS here
}
- CSS offers several ways to identify which elements to apply a style to using a selector.
- You may combine selectors to further specify which elements to apply styling to.
13. JavaScript
JavaScript is a programming language that provides instructions to a web browser.
- JavaScript is NOT a server-side language. It is a scripting language.
- Is NOT related to Java
- Designed to manipulate a web page
- Use the <script>...</script> tag
- The type attribute is optional
- You can also include an external .js file <script src="
file.js ">...</script>
javaScript Datatypes
- String: represents a list of characters ex: "abc", '1nd*3j2n'
- Number: represents an integer or decimal value ex: 100, 12.0383
- Boolean: represents either true or false ex: true or false
- Object: represents a group of key/value pairs
- Date: represents date/time Date.now()
- Array: represents a collection of data ex: var arr = [1,'232', 582];
- undefined: represents a value not yet defined
- null: represents an empty value
ex: Cat = {
name:'Mittens',
age:2,
}
- A variable is a temporary storage unit that stores data
- var variableName;
- declaration is the process of creating a named variable
- follow camelCase convention
- names must begin with letter, _,or $
- numbers, and Unicode escape sequences can be used after the first character
- names are case-sensitive: numOfTurns is not equal to NumOfTurns
- Use the equals sign (=) to assign a value to a variable
var a;
a = 25;
a = 13; //the variable has a new value
a = 'hello'; //the variable has a new data type Re-Assigning to Null
- To set the value of a variable to an empty value use null var a = 23;
a = null;